qcacld-3.0: Add spatial reuse IE
Add spatial reuse IE Change-Id: I84f6963dff46283599ed1d9cd714b44a573a8c4c CRs-Fixed: 3213416
This commit is contained in:
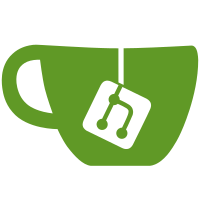
committed by
Madan Koyyalamudi
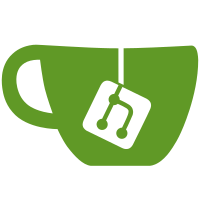
parent
64d47b8e70
commit
b7fe086a66
@@ -3237,6 +3237,35 @@ IE he_op (EID_EXTN_ID_ELEMENT) OUI (0x24)
|
||||
};
|
||||
}
|
||||
|
||||
IE spatial_reuse (EID_EXTN_ID_ELEMENT) OUI (0x27)
|
||||
{
|
||||
{
|
||||
psr_disallow: 1;
|
||||
non_srg_pd_sr_disallow: 1;
|
||||
non_srg_offset_present: 1;
|
||||
srg_info_present: 1;
|
||||
sr_value15_allow: 1;
|
||||
reserved: 3;
|
||||
}
|
||||
OPTIONAL UNION non_srg_offset (DISCRIMINATOR non_srg_offset_present)
|
||||
{
|
||||
info (non_srg_offset_present IS 1)
|
||||
{
|
||||
non_srg_pd_max_offset, 1;
|
||||
}
|
||||
};
|
||||
OPTIONAL UNION srg_info (DISCRIMINATOR srg_info_present)
|
||||
{
|
||||
info (srg_info_present IS 1)
|
||||
{
|
||||
srg_pd_min_offset, 1;
|
||||
srg_pd_max_offset, 1;
|
||||
srg_color[8];
|
||||
srg_partial_bssid[8];
|
||||
}
|
||||
};
|
||||
}
|
||||
|
||||
IE he_6ghz_band_cap (EID_EXTN_ID_ELEMENT) OUI (0x3B)
|
||||
{
|
||||
{ // capabilities_information
|
||||
@@ -3831,6 +3860,7 @@ FRAME Beacon // C.f. Sec. 7.2.3.1
|
||||
OPTIE esp_information;
|
||||
OPTIE he_cap;
|
||||
OPTIE he_op;
|
||||
OPTIE spatial_reuse;
|
||||
OPTIE bss_color_change;
|
||||
OPTIE mu_edca_param_set;
|
||||
OPTIE he_6ghz_band_cap;
|
||||
@@ -3935,6 +3965,7 @@ FRAME Beacon2
|
||||
OPTIE esp_information;
|
||||
OPTIE he_cap;
|
||||
OPTIE he_op;
|
||||
OPTIE spatial_reuse;
|
||||
OPTIE bss_color_change;
|
||||
OPTIE mu_edca_param_set;
|
||||
OPTIE he_6ghz_band_cap;
|
||||
@@ -4013,6 +4044,7 @@ FRAME BeaconIEs
|
||||
OPTIE esp_information;
|
||||
OPTIE he_cap;
|
||||
OPTIE he_op;
|
||||
OPTIE spatial_reuse;
|
||||
OPTIE bss_color_change;
|
||||
OPTIE mu_edca_param_set;
|
||||
OPTIE he_6ghz_band_cap;
|
||||
@@ -4129,6 +4161,7 @@ FRAME AssocResponse // 7.2.3.5
|
||||
OPTIE fils_hlp_container;
|
||||
OPTIE he_cap;
|
||||
OPTIE he_op;
|
||||
OPTIE spatial_reuse;
|
||||
OPTIE bss_color_change;
|
||||
OPTIE mu_edca_param_set;
|
||||
OPTIE he_6ghz_band_cap;
|
||||
@@ -4227,6 +4260,7 @@ FRAME ReAssocResponse // 7.2.3.7
|
||||
OPTIE OperatingMode;
|
||||
OPTIE he_cap;
|
||||
OPTIE he_op;
|
||||
OPTIE spatial_reuse;
|
||||
OPTIE bss_color_change;
|
||||
OPTIE mu_edca_param_set;
|
||||
OPTIE he_6ghz_band_cap;
|
||||
@@ -4313,6 +4347,7 @@ FRAME ProbeResponse // 7.2.3.9
|
||||
OPTIE esp_information;
|
||||
OPTIE he_cap;
|
||||
OPTIE he_op;
|
||||
OPTIE spatial_reuse;
|
||||
OPTIE bss_color_change;
|
||||
OPTIE mu_edca_param_set;
|
||||
OPTIE he_6ghz_band_cap;
|
||||
|
@@ -27,7 +27,7 @@
|
||||
*
|
||||
*
|
||||
* This file was automatically generated by 'framesc'
|
||||
* Mon Jun 13 10:50:26 2022 from the following file(s):
|
||||
* Thu Jun 16 20:13:08 2022 from the following file(s):
|
||||
*
|
||||
* dot11f.frms
|
||||
*
|
||||
@@ -10337,6 +10337,63 @@ uint32_t dot11f_get_packed_ie_sec_chan_offset_ele(
|
||||
}; /* End extern "C". */
|
||||
#endif /* C++ */
|
||||
|
||||
/* EID 255 (0xff) Extended EID 39 (0x27) */
|
||||
typedef struct sDot11fIEspatial_reuse {
|
||||
uint8_t present;
|
||||
uint8_t psr_disallow:1;
|
||||
uint8_t non_srg_pd_sr_disallow:1;
|
||||
uint8_t non_srg_offset_present:1;
|
||||
uint8_t srg_info_present:1;
|
||||
uint8_t sr_value15_allow:1;
|
||||
uint8_t reserved:3;
|
||||
union {
|
||||
struct {
|
||||
uint8_t non_srg_pd_max_offset;
|
||||
} info; /* non_srg_offset_present = 1 */
|
||||
} non_srg_offset;
|
||||
union {
|
||||
struct {
|
||||
uint8_t srg_pd_min_offset;
|
||||
uint8_t srg_pd_max_offset;
|
||||
uint8_t srg_color[8];
|
||||
uint8_t srg_partial_bssid[8];
|
||||
} info; /* srg_info_present = 1 */
|
||||
} srg_info;
|
||||
} tDot11fIEspatial_reuse;
|
||||
|
||||
#define DOT11F_EID_SPATIAL_REUSE (255)
|
||||
|
||||
/* N.B. These #defines do *not* include the EID & length */
|
||||
#define DOT11F_IE_SPATIAL_REUSE_MIN_LEN (1)
|
||||
|
||||
#define DOT11F_IE_SPATIAL_REUSE_MAX_LEN (20)
|
||||
|
||||
#ifdef __cplusplus
|
||||
extern "C" {
|
||||
#endif /* C++ */
|
||||
__must_check uint32_t dot11f_unpack_ie_spatial_reuse(
|
||||
tpAniSirGlobal,
|
||||
uint8_t *,
|
||||
uint8_t,
|
||||
tDot11fIEspatial_reuse *,
|
||||
bool);
|
||||
|
||||
uint32_t dot11f_pack_ie_spatial_reuse(
|
||||
tpAniSirGlobal,
|
||||
tDot11fIEspatial_reuse *,
|
||||
uint8_t *,
|
||||
uint32_t,
|
||||
uint32_t*);
|
||||
|
||||
uint32_t dot11f_get_packed_ie_spatial_reuse(
|
||||
tpAniSirGlobal,
|
||||
tDot11fIEspatial_reuse *,
|
||||
uint32_t*);
|
||||
|
||||
#ifdef __cplusplus
|
||||
}; /* End extern "C". */
|
||||
#endif /* C++ */
|
||||
|
||||
/* EID 221 (0xdd) {OUI 0x00, 0x90, 0x4c, 0x04} */
|
||||
typedef struct sDot11fIEvendor_vht_ie {
|
||||
uint8_t present;
|
||||
@@ -10551,6 +10608,7 @@ typedef struct sDot11fAssocResponse{
|
||||
tDot11fIEfils_hlp_container fils_hlp_container;
|
||||
tDot11fIEhe_cap he_cap;
|
||||
tDot11fIEhe_op he_op;
|
||||
tDot11fIEspatial_reuse spatial_reuse;
|
||||
tDot11fIEbss_color_change bss_color_change;
|
||||
tDot11fIEmu_edca_param_set mu_edca_param_set;
|
||||
tDot11fIEhe_6ghz_band_cap he_6ghz_band_cap;
|
||||
@@ -10679,6 +10737,7 @@ typedef struct sDot11fBeacon{
|
||||
tDot11fIEesp_information esp_information;
|
||||
tDot11fIEhe_cap he_cap;
|
||||
tDot11fIEhe_op he_op;
|
||||
tDot11fIEspatial_reuse spatial_reuse;
|
||||
tDot11fIEbss_color_change bss_color_change;
|
||||
tDot11fIEmu_edca_param_set mu_edca_param_set;
|
||||
tDot11fIEhe_6ghz_band_cap he_6ghz_band_cap;
|
||||
@@ -10788,6 +10847,7 @@ typedef struct sDot11fBeacon2{
|
||||
tDot11fIEesp_information esp_information;
|
||||
tDot11fIEhe_cap he_cap;
|
||||
tDot11fIEhe_op he_op;
|
||||
tDot11fIEspatial_reuse spatial_reuse;
|
||||
tDot11fIEbss_color_change bss_color_change;
|
||||
tDot11fIEmu_edca_param_set mu_edca_param_set;
|
||||
tDot11fIEhe_6ghz_band_cap he_6ghz_band_cap;
|
||||
@@ -10877,6 +10937,7 @@ typedef struct sDot11fBeaconIEs{
|
||||
tDot11fIEesp_information esp_information;
|
||||
tDot11fIEhe_cap he_cap;
|
||||
tDot11fIEhe_op he_op;
|
||||
tDot11fIEspatial_reuse spatial_reuse;
|
||||
tDot11fIEbss_color_change bss_color_change;
|
||||
tDot11fIEmu_edca_param_set mu_edca_param_set;
|
||||
tDot11fIEhe_6ghz_band_cap he_6ghz_band_cap;
|
||||
@@ -11309,6 +11370,7 @@ typedef struct sDot11fProbeResponse{
|
||||
tDot11fIEesp_information esp_information;
|
||||
tDot11fIEhe_cap he_cap;
|
||||
tDot11fIEhe_op he_op;
|
||||
tDot11fIEspatial_reuse spatial_reuse;
|
||||
tDot11fIEbss_color_change bss_color_change;
|
||||
tDot11fIEmu_edca_param_set mu_edca_param_set;
|
||||
tDot11fIEhe_6ghz_band_cap he_6ghz_band_cap;
|
||||
@@ -11531,6 +11593,7 @@ typedef struct sDot11fReAssocResponse{
|
||||
tDot11fIEOperatingMode OperatingMode;
|
||||
tDot11fIEhe_cap he_cap;
|
||||
tDot11fIEhe_op he_op;
|
||||
tDot11fIEspatial_reuse spatial_reuse;
|
||||
tDot11fIEbss_color_change bss_color_change;
|
||||
tDot11fIEmu_edca_param_set mu_edca_param_set;
|
||||
tDot11fIEhe_6ghz_band_cap he_6ghz_band_cap;
|
||||
|
@@ -25,7 +25,7 @@
|
||||
*
|
||||
*
|
||||
* This file was automatically generated by 'framesc'
|
||||
* Mon Jun 13 10:50:26 2022 from the following file(s):
|
||||
* Thu Jun 16 20:13:08 2022 from the following file(s):
|
||||
*
|
||||
* dot11f.frms
|
||||
*
|
||||
@@ -10487,6 +10487,87 @@ uint32_t dot11f_unpack_ie_sec_chan_offset_ele(tpAniSirGlobal pCtx,
|
||||
|
||||
#define SigIesec_chan_offset_ele (0x00a0)
|
||||
|
||||
uint32_t dot11f_unpack_ie_spatial_reuse(tpAniSirGlobal pCtx,
|
||||
uint8_t *pBuf,
|
||||
uint8_t ielen,
|
||||
tDot11fIEspatial_reuse *pDst,
|
||||
bool append_ie)
|
||||
{
|
||||
uint32_t status = DOT11F_PARSE_SUCCESS;
|
||||
uint8_t tmp111__;
|
||||
(void)pBuf; (void)ielen; /* Shutup the compiler */
|
||||
if (pDst->present)
|
||||
return DOT11F_DUPLICATE_IE;
|
||||
pDst->present = 1;
|
||||
if (unlikely(ielen < 1)) {
|
||||
pDst->present = 0;
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
}
|
||||
|
||||
tmp111__ = *pBuf;
|
||||
pBuf += 1;
|
||||
ielen -= 1;
|
||||
pDst->psr_disallow = tmp111__ >> 0 & 0x1;
|
||||
pDst->non_srg_pd_sr_disallow = tmp111__ >> 1 & 0x1;
|
||||
pDst->non_srg_offset_present = tmp111__ >> 2 & 0x1;
|
||||
pDst->srg_info_present = tmp111__ >> 3 & 0x1;
|
||||
pDst->sr_value15_allow = tmp111__ >> 4 & 0x1;
|
||||
pDst->reserved = tmp111__ >> 5 & 0x7;
|
||||
switch (pDst->non_srg_offset_present) {
|
||||
case 1:
|
||||
if (unlikely(ielen < 1)) {
|
||||
pDst->present = 0;
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
}
|
||||
|
||||
pDst->non_srg_offset.info.non_srg_pd_max_offset = *pBuf;
|
||||
pBuf += 1;
|
||||
ielen -= (uint8_t)1;
|
||||
break;
|
||||
}
|
||||
switch (pDst->srg_info_present) {
|
||||
case 1:
|
||||
if (unlikely(ielen < 1)) {
|
||||
pDst->present = 0;
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
}
|
||||
|
||||
pDst->srg_info.info.srg_pd_min_offset = *pBuf;
|
||||
pBuf += 1;
|
||||
ielen -= (uint8_t)1;
|
||||
if (unlikely(ielen < 1)) {
|
||||
pDst->present = 0;
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
}
|
||||
|
||||
pDst->srg_info.info.srg_pd_max_offset = *pBuf;
|
||||
pBuf += 1;
|
||||
ielen -= (uint8_t)1;
|
||||
if (unlikely(ielen < 8)) {
|
||||
pDst->present = 0;
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
}
|
||||
|
||||
DOT11F_MEMCPY(pCtx, pDst->srg_info.info.srg_color, pBuf, 8);
|
||||
pBuf += 8;
|
||||
ielen -= (uint8_t)8;
|
||||
if (unlikely(ielen < 8)) {
|
||||
pDst->present = 0;
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
}
|
||||
|
||||
DOT11F_MEMCPY(pCtx, pDst->srg_info.info.srg_partial_bssid,
|
||||
pBuf, 8);
|
||||
pBuf += 8;
|
||||
ielen -= (uint8_t)8;
|
||||
break;
|
||||
}
|
||||
(void)pCtx;
|
||||
return status;
|
||||
} /* End dot11f_unpack_ie_spatial_reuse. */
|
||||
|
||||
#define SigIespatial_reuse (0x00a1)
|
||||
|
||||
|
||||
static const tFFDefn FFS_vendor_vht_ie[] = {
|
||||
{ NULL, 0, 0, 0,},
|
||||
@@ -10534,7 +10615,7 @@ uint32_t dot11f_unpack_ie_vendor_vht_ie(tpAniSirGlobal pCtx,
|
||||
return status;
|
||||
} /* End dot11f_unpack_ie_vendor_vht_ie. */
|
||||
|
||||
#define SigIevendor_vht_ie (0x00a1)
|
||||
#define SigIevendor_vht_ie (0x00a2)
|
||||
|
||||
|
||||
static const tFFDefn FFS_AddTSRequest[] = {
|
||||
@@ -10931,6 +11012,10 @@ static const tIEDefn IES_AssocResponse[] = {
|
||||
{ offsetof(tDot11fAssocResponse, he_op), offsetof(tDot11fIEhe_op,
|
||||
present), 0, "he_op", 0, 8, 17, SigIehe_op, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_HE_OP, 36, 0, },
|
||||
{ offsetof(tDot11fAssocResponse, spatial_reuse),
|
||||
offsetof(tDot11fIEspatial_reuse, present), 0, "spatial_reuse",
|
||||
0, 3, 22, SigIespatial_reuse, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_SPATIAL_REUSE, 39, 0, },
|
||||
{ offsetof(tDot11fAssocResponse, bss_color_change),
|
||||
offsetof(tDot11fIEbss_color_change, present), 0, "bss_color_change",
|
||||
0, 4, 4, SigIebss_color_change, {0, 0, 0, 0, 0},
|
||||
@@ -11239,6 +11324,10 @@ static const tIEDefn IES_Beacon[] = {
|
||||
{ offsetof(tDot11fBeacon, he_op), offsetof(tDot11fIEhe_op, present), 0,
|
||||
"he_op", 0, 8, 17, SigIehe_op, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_HE_OP, 36, 0, },
|
||||
{ offsetof(tDot11fBeacon, spatial_reuse),
|
||||
offsetof(tDot11fIEspatial_reuse, present), 0, "spatial_reuse",
|
||||
0, 3, 22, SigIespatial_reuse, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_SPATIAL_REUSE, 39, 0, },
|
||||
{ offsetof(tDot11fBeacon, bss_color_change),
|
||||
offsetof(tDot11fIEbss_color_change, present), 0, "bss_color_change",
|
||||
0, 4, 4, SigIebss_color_change, {0, 0, 0, 0, 0},
|
||||
@@ -11491,6 +11580,10 @@ static const tIEDefn IES_Beacon2[] = {
|
||||
{ offsetof(tDot11fBeacon2, he_op), offsetof(tDot11fIEhe_op, present), 0,
|
||||
"he_op", 0, 8, 17, SigIehe_op, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_HE_OP, 36, 0, },
|
||||
{ offsetof(tDot11fBeacon2, spatial_reuse),
|
||||
offsetof(tDot11fIEspatial_reuse, present), 0, "spatial_reuse",
|
||||
0, 3, 22, SigIespatial_reuse, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_SPATIAL_REUSE, 39, 0, },
|
||||
{ offsetof(tDot11fBeacon2, bss_color_change),
|
||||
offsetof(tDot11fIEbss_color_change, present), 0, "bss_color_change",
|
||||
0, 4, 4, SigIebss_color_change, {0, 0, 0, 0, 0},
|
||||
@@ -11734,6 +11827,10 @@ static const tIEDefn IES_BeaconIEs[] = {
|
||||
{ offsetof(tDot11fBeaconIEs, he_op), offsetof(tDot11fIEhe_op, present), 0,
|
||||
"he_op", 0, 8, 17, SigIehe_op, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_HE_OP, 36, 0, },
|
||||
{ offsetof(tDot11fBeaconIEs, spatial_reuse),
|
||||
offsetof(tDot11fIEspatial_reuse, present), 0, "spatial_reuse",
|
||||
0, 3, 22, SigIespatial_reuse, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_SPATIAL_REUSE, 39, 0, },
|
||||
{ offsetof(tDot11fBeaconIEs, bss_color_change),
|
||||
offsetof(tDot11fIEbss_color_change, present), 0, "bss_color_change",
|
||||
0, 4, 4, SigIebss_color_change, {0, 0, 0, 0, 0},
|
||||
@@ -12414,6 +12511,10 @@ static const tIEDefn IES_ProbeResponse[] = {
|
||||
{ offsetof(tDot11fProbeResponse, he_op), offsetof(tDot11fIEhe_op,
|
||||
present), 0, "he_op", 0, 8, 17, SigIehe_op, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_HE_OP, 36, 0, },
|
||||
{ offsetof(tDot11fProbeResponse, spatial_reuse),
|
||||
offsetof(tDot11fIEspatial_reuse, present), 0, "spatial_reuse",
|
||||
0, 3, 22, SigIespatial_reuse, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_SPATIAL_REUSE, 39, 0, },
|
||||
{ offsetof(tDot11fProbeResponse, bss_color_change),
|
||||
offsetof(tDot11fIEbss_color_change, present), 0, "bss_color_change",
|
||||
0, 4, 4, SigIebss_color_change, {0, 0, 0, 0, 0},
|
||||
@@ -12875,6 +12976,10 @@ static const tIEDefn IES_ReAssocResponse[] = {
|
||||
{ offsetof(tDot11fReAssocResponse, he_op), offsetof(tDot11fIEhe_op,
|
||||
present), 0, "he_op", 0, 8, 17, SigIehe_op, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_HE_OP, 36, 0, },
|
||||
{ offsetof(tDot11fReAssocResponse, spatial_reuse),
|
||||
offsetof(tDot11fIEspatial_reuse, present), 0, "spatial_reuse",
|
||||
0, 3, 22, SigIespatial_reuse, {0, 0, 0, 0, 0},
|
||||
0, DOT11F_EID_SPATIAL_REUSE, 39, 0, },
|
||||
{ offsetof(tDot11fReAssocResponse, bss_color_change),
|
||||
offsetof(tDot11fIEbss_color_change, present), 0, "bss_color_change",
|
||||
0, 4, 4, SigIebss_color_change, {0, 0, 0, 0, 0},
|
||||
@@ -16001,6 +16106,16 @@ static uint32_t unpack_core(tpAniSirGlobal pCtx,
|
||||
countOffset),
|
||||
append_ie);
|
||||
break;
|
||||
case SigIespatial_reuse:
|
||||
status |=
|
||||
dot11f_unpack_ie_spatial_reuse(
|
||||
pCtx, pBufRemaining, len,
|
||||
(tDot11fIEspatial_reuse *)
|
||||
(pFrm + pIe->offset +
|
||||
sizeof(tDot11fIEspatial_reuse) *
|
||||
countOffset),
|
||||
append_ie);
|
||||
break;
|
||||
case SigIevendor_vht_ie:
|
||||
status |=
|
||||
dot11f_unpack_ie_vendor_vht_ie(
|
||||
@@ -17600,6 +17715,40 @@ uint32_t dot11f_get_packed_ie_reduced_neighbor_report(tpAniSirGlobal pCtx,
|
||||
return status;
|
||||
} /* End dot11f_get_packed_ie_reduced_neighbor_report. */
|
||||
|
||||
uint32_t dot11f_get_packed_ie_spatial_reuse(tpAniSirGlobal pCtx,
|
||||
tDot11fIEspatial_reuse *pIe,
|
||||
uint32_t *pnNeeded)
|
||||
{
|
||||
uint32_t status = DOT11F_PARSE_SUCCESS;
|
||||
(void)pCtx;
|
||||
while (pIe->present) {
|
||||
*pnNeeded += 1;
|
||||
if (pIe->non_srg_offset_present) {
|
||||
switch (pIe->non_srg_offset_present) {
|
||||
case 1:
|
||||
*pnNeeded += 1;
|
||||
break;
|
||||
}
|
||||
} else {
|
||||
break;
|
||||
}
|
||||
if (pIe->srg_info_present) {
|
||||
switch (pIe->srg_info_present) {
|
||||
case 1:
|
||||
*pnNeeded += 1;
|
||||
*pnNeeded += 1;
|
||||
*pnNeeded += 8;
|
||||
*pnNeeded += 8;
|
||||
break;
|
||||
}
|
||||
} else {
|
||||
break;
|
||||
}
|
||||
break;
|
||||
}
|
||||
return status;
|
||||
} /* End dot11f_get_packed_ie_spatial_reuse. */
|
||||
|
||||
uint32_t dot11f_get_packed_ie_vendor_vht_ie(tpAniSirGlobal pCtx,
|
||||
tDot11fIEvendor_vht_ie *pIe, uint32_t *pnNeeded)
|
||||
{
|
||||
@@ -19422,6 +19571,14 @@ static uint32_t get_packed_size_core(tpAniSirGlobal pCtx,
|
||||
(pFrm + pIe->offset + offset * i))->
|
||||
present;
|
||||
break;
|
||||
case SigIespatial_reuse:
|
||||
offset = sizeof(tDot11fIEspatial_reuse);
|
||||
status |=
|
||||
dot11f_get_packed_ie_spatial_reuse(
|
||||
pCtx, (tDot11fIEspatial_reuse *)
|
||||
(pFrm + pIe->offset + offset * i),
|
||||
pnNeeded);
|
||||
break;
|
||||
case SigIevendor_vht_ie:
|
||||
offset = sizeof(tDot11fIEvendor_vht_ie);
|
||||
status |=
|
||||
@@ -29799,6 +29956,87 @@ uint32_t dot11f_pack_ie_sec_chan_offset_ele(tpAniSirGlobal pCtx,
|
||||
return DOT11F_PARSE_SUCCESS;
|
||||
} /* End dot11f_pack_ie_sec_chan_offset_ele. */
|
||||
|
||||
uint32_t dot11f_pack_ie_spatial_reuse(tpAniSirGlobal pCtx,
|
||||
tDot11fIEspatial_reuse *pSrc,
|
||||
uint8_t *pBuf,
|
||||
uint32_t nBuf,
|
||||
uint32_t *pnConsumed)
|
||||
{
|
||||
uint8_t *pIeLen = 0;
|
||||
uint32_t nConsumedOnEntry = *pnConsumed;
|
||||
uint32_t nNeeded = 0U;
|
||||
uint8_t tmp239__;
|
||||
uint32_t status = DOT11F_PARSE_SUCCESS;
|
||||
|
||||
status = dot11f_get_packed_ie_spatial_reuse(pCtx, pSrc, &nNeeded);
|
||||
if (!DOT11F_SUCCEEDED(status))
|
||||
return status;
|
||||
while (pSrc->present) {
|
||||
if (nNeeded > nBuf)
|
||||
return DOT11F_BUFFER_OVERFLOW;
|
||||
*pBuf = 255;
|
||||
++pBuf; ++(*pnConsumed);
|
||||
pIeLen = pBuf;
|
||||
++pBuf; ++(*pnConsumed);
|
||||
*pBuf = 39;
|
||||
++pBuf; ++(*pnConsumed);
|
||||
tmp239__ = 0U;
|
||||
tmp239__ |= (pSrc->psr_disallow << 0);
|
||||
tmp239__ |= (pSrc->non_srg_pd_sr_disallow << 1);
|
||||
tmp239__ |= (pSrc->non_srg_offset_present << 2);
|
||||
tmp239__ |= (pSrc->srg_info_present << 3);
|
||||
tmp239__ |= (pSrc->sr_value15_allow << 4);
|
||||
tmp239__ |= (pSrc->reserved << 5);
|
||||
if (unlikely(nBuf < 1))
|
||||
return DOT11F_INCOMPLETE_IE;
|
||||
|
||||
*pBuf = tmp239__;
|
||||
*pnConsumed += 1;
|
||||
pBuf += 1;
|
||||
nBuf -= 1;
|
||||
if (pSrc->non_srg_offset_present) {
|
||||
switch (pSrc->non_srg_offset_present) {
|
||||
case 1:
|
||||
*pBuf = pSrc->non_srg_offset.info.non_srg_pd_max_offset;
|
||||
*pnConsumed += 1;
|
||||
pBuf += 1;
|
||||
break;
|
||||
}
|
||||
} else {
|
||||
break;
|
||||
}
|
||||
if (pSrc->srg_info_present) {
|
||||
switch (pSrc->srg_info_present) {
|
||||
case 1:
|
||||
*pBuf = pSrc->srg_info.info.srg_pd_min_offset;
|
||||
*pnConsumed += 1;
|
||||
pBuf += 1;
|
||||
*pBuf = pSrc->srg_info.info.srg_pd_max_offset;
|
||||
*pnConsumed += 1;
|
||||
pBuf += 1;
|
||||
DOT11F_MEMCPY(pCtx, pBuf,
|
||||
pSrc->srg_info.info.srg_color, 8);
|
||||
*pnConsumed += 8;
|
||||
pBuf += 8;
|
||||
DOT11F_MEMCPY(
|
||||
pCtx, pBuf,
|
||||
pSrc->srg_info.info.srg_partial_bssid,
|
||||
8);
|
||||
*pnConsumed += 8;
|
||||
/* fieldsEndFlag = 1 */
|
||||
break;
|
||||
}
|
||||
} else {
|
||||
break;
|
||||
}
|
||||
break;
|
||||
}
|
||||
(void)pCtx;
|
||||
if (pIeLen)
|
||||
*pIeLen = *pnConsumed - nConsumedOnEntry - 2;
|
||||
return status;
|
||||
} /* End dot11f_pack_ie_spatial_reuse. */
|
||||
|
||||
uint32_t dot11f_pack_ie_vendor_vht_ie(tpAniSirGlobal pCtx,
|
||||
tDot11fIEvendor_vht_ie *pSrc,
|
||||
uint8_t *pBuf,
|
||||
@@ -32181,6 +32419,14 @@ static uint32_t pack_core(tpAniSirGlobal pCtx,
|
||||
sizeof(tDot11fIEsec_chan_offset_ele) * i),
|
||||
pBufRemaining, nBufRemaining, &len);
|
||||
break;
|
||||
case SigIespatial_reuse:
|
||||
status |=
|
||||
dot11f_pack_ie_spatial_reuse(
|
||||
pCtx, (tDot11fIEspatial_reuse *)
|
||||
(pSrc + pIe->offset +
|
||||
sizeof(tDot11fIEspatial_reuse) * i),
|
||||
pBufRemaining, nBufRemaining, &len);
|
||||
break;
|
||||
case SigIevendor_vht_ie:
|
||||
status |=
|
||||
dot11f_pack_ie_vendor_vht_ie(
|
||||
|
Reference in New Issue
Block a user