qcacld-3.0: Disable beamformer for single chain
If there is single chain support then driver should disable beamformer instead of enable As part of fix, check the number of chains by intersecting the firmware capability and ini config. If it is 1 then set su_bformer, mu_bformer and num_soundingdim as 0 Change-Id: I84434c741cbf774f368657b2c57668f6e7b99991 CRs-Fixed: 3003257
This commit is contained in:
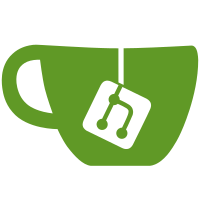
committed by
Madan Koyyalamudi
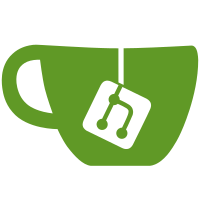
parent
5842e66a2b
commit
6209487c3c
@@ -2723,6 +2723,8 @@ int hdd_update_tgt_cfg(hdd_handle_t hdd_handle, struct wma_tgt_cfg *cfg)
|
||||
|
||||
hdd_update_tgt_ht_cap(hdd_ctx, &cfg->ht_cap);
|
||||
|
||||
sme_update_bfer_caps_as_per_nss_chains(hdd_ctx->mac_handle, cfg);
|
||||
|
||||
hdd_update_tgt_vht_cap(hdd_ctx, &cfg->vht_cap);
|
||||
if (cfg->services.en_11ax &&
|
||||
(hdd_ctx->config->dot11Mode == eHDD_DOT11_MODE_AUTO ||
|
||||
|
@@ -354,6 +354,20 @@ sme_nss_chains_update(mac_handle_t mac_handle,
|
||||
struct wlan_mlme_nss_chains *user_cfg,
|
||||
uint8_t vdev_id);
|
||||
|
||||
/**
|
||||
* sme_update_bfer_caps_as_per_nss_chains() - Update beamformer caps as per nss
|
||||
* chains.
|
||||
* @mac_handle: The handle returned by mac_open
|
||||
* @cfg: wma target config
|
||||
*
|
||||
* This API will update beamformer capability as per nss chains
|
||||
*
|
||||
* Return: None
|
||||
*/
|
||||
void
|
||||
sme_update_bfer_caps_as_per_nss_chains(mac_handle_t mac_handle,
|
||||
struct wma_tgt_cfg *cfg);
|
||||
|
||||
/**
|
||||
* sme_vdev_create() - Create vdev for given persona
|
||||
* @mac_handle: The handle returned by mac_open
|
||||
|
@@ -4577,6 +4577,64 @@ release_ref:
|
||||
return status;
|
||||
}
|
||||
|
||||
#ifdef WLAN_FEATURE_11AX
|
||||
static void sme_update_bfer_he_cap(struct wma_tgt_cfg *cfg)
|
||||
{
|
||||
cfg->he_cap_5g.su_beamformer = 0;
|
||||
}
|
||||
#else
|
||||
static void sme_update_bfer_he_cap(struct wma_tgt_cfg *cfg)
|
||||
{
|
||||
}
|
||||
#endif
|
||||
|
||||
#ifdef WLAN_FEATURE_11BE
|
||||
void sme_update_bfer_eht_cap(struct wma_tgt_cfg *cfg)
|
||||
{
|
||||
cfg->eht_cap_5g.su_beamformer = 0;
|
||||
}
|
||||
#else
|
||||
static void sme_update_bfer_eht_cap(struct wma_tgt_cfg *cfg)
|
||||
{
|
||||
}
|
||||
#endif
|
||||
|
||||
void sme_update_bfer_caps_as_per_nss_chains(mac_handle_t mac_handle,
|
||||
struct wma_tgt_cfg *cfg)
|
||||
{
|
||||
uint8_t max_supported_tx_chains = MAX_VDEV_CHAINS;
|
||||
struct mac_context *mac_ctx = MAC_CONTEXT(mac_handle);
|
||||
struct wlan_mlme_nss_chains *nss_chains_ini_cfg =
|
||||
&mac_ctx->mlme_cfg->nss_chains_ini_cfg;
|
||||
uint8_t ini_tx_chains;
|
||||
|
||||
ini_tx_chains = GET_VDEV_NSS_CHAIN(
|
||||
nss_chains_ini_cfg->num_tx_chains[NSS_CHAINS_BAND_5GHZ],
|
||||
SAP_NSS_CHAINS_SHIFT);
|
||||
|
||||
max_supported_tx_chains =
|
||||
mac_ctx->fw_chain_cfg.max_tx_chains_5g;
|
||||
|
||||
max_supported_tx_chains = QDF_MIN(ini_tx_chains,
|
||||
max_supported_tx_chains);
|
||||
if (!max_supported_tx_chains)
|
||||
return;
|
||||
|
||||
if (max_supported_tx_chains == 1) {
|
||||
sme_debug("ini support %d and firmware support %d",
|
||||
ini_tx_chains,
|
||||
mac_ctx->fw_chain_cfg.max_tx_chains_5g);
|
||||
if (mac_ctx->fw_chain_cfg.max_tx_chains_5g == 1) {
|
||||
cfg->vht_cap.vht_su_bformer = 0;
|
||||
sme_update_bfer_he_cap(cfg);
|
||||
sme_update_bfer_eht_cap(cfg);
|
||||
}
|
||||
mac_ctx->mlme_cfg->vht_caps.vht_cap_info.su_bformer = 0;
|
||||
mac_ctx->mlme_cfg->vht_caps.vht_cap_info.num_soundingdim = 0;
|
||||
mac_ctx->mlme_cfg->vht_caps.vht_cap_info.mu_bformer = 0;
|
||||
}
|
||||
}
|
||||
|
||||
QDF_STATUS sme_vdev_post_vdev_create_setup(mac_handle_t mac_handle,
|
||||
struct wlan_objmgr_vdev *vdev)
|
||||
{
|
||||
|
Reference in New Issue
Block a user