qcacld-3.0: Fill Current MAC Frequency Range
During wma_pdev_hw_mode_transition_evt_handler() get the freq range from mac_freq_mapping[] present in wmi_pdev_hw_mode_transition_event_fixed_param and pass till policy_mgr_hw_mode_transition_cb() and update cur_mac_freq_range[MAX_MAC] from it. if no freq range provided (legacy chipsets) then use freq_range_caps[MODE_HW_MAX][MAX_MAC] to fill the range depending on the new HW mode. Change-Id: I145d48052ce37724b0f697c19a1041f5a908cb54 CRs-Fixed: 3044976
Dieser Commit ist enthalten in:
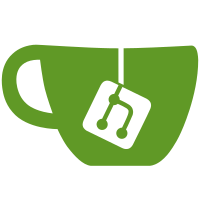
committet von
Madan Koyyalamudi
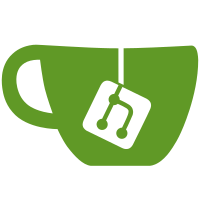
Ursprung
21ea2c1156
Commit
b133ed84cb
@@ -2463,6 +2463,8 @@ QDF_STATUS policy_mgr_check_conn_with_mode_and_vdev_id(
|
||||
* @new_hw_mode_index: New HW mode index
|
||||
* @num_vdev_mac_entries: Number of vdev-mac id mapping that follows
|
||||
* @vdev_mac_map: vdev-mac id map. This memory will be freed by the caller.
|
||||
* @num_mac_freq: Number of pdev freq maping that follows
|
||||
* @mac_freq_range: mac_freq_range mapping
|
||||
* So, make local copy if needed.
|
||||
*
|
||||
* Provides the old and new HW mode index set by the FW
|
||||
@@ -2473,6 +2475,8 @@ void policy_mgr_hw_mode_transition_cb(uint32_t old_hw_mode_index,
|
||||
uint32_t new_hw_mode_index,
|
||||
uint32_t num_vdev_mac_entries,
|
||||
struct policy_mgr_vdev_mac_map *vdev_mac_map,
|
||||
uint32_t num_mac_freq,
|
||||
struct policy_mgr_pdev_mac_freq_map *mac_freq_range,
|
||||
struct wlan_objmgr_psoc *context);
|
||||
|
||||
/**
|
||||
|
@@ -45,6 +45,8 @@ void policy_mgr_hw_mode_transition_cb(uint32_t old_hw_mode_index,
|
||||
uint32_t new_hw_mode_index,
|
||||
uint32_t num_vdev_mac_entries,
|
||||
struct policy_mgr_vdev_mac_map *vdev_mac_map,
|
||||
uint32_t num_mac_freq,
|
||||
struct policy_mgr_pdev_mac_freq_map *mac_freq_range,
|
||||
struct wlan_objmgr_psoc *context)
|
||||
{
|
||||
QDF_STATUS status;
|
||||
@@ -66,6 +68,13 @@ void policy_mgr_hw_mode_transition_cb(uint32_t old_hw_mode_index,
|
||||
policy_mgr_debug("old_hw_mode_index=%d, new_hw_mode_index=%d",
|
||||
old_hw_mode_index, new_hw_mode_index);
|
||||
|
||||
if (mac_freq_range)
|
||||
for (i = 0; i < num_mac_freq; i++)
|
||||
policy_mgr_debug("pdev_id:%d start_freq:%d end_freq %d",
|
||||
mac_freq_range[i].pdev_id,
|
||||
mac_freq_range[i].start_freq,
|
||||
mac_freq_range[i].end_freq);
|
||||
|
||||
for (i = 0; i < num_vdev_mac_entries; i++)
|
||||
policy_mgr_debug("vdev_id:%d mac_id:%d",
|
||||
vdev_mac_map[i].vdev_id,
|
||||
@@ -90,8 +99,8 @@ void policy_mgr_hw_mode_transition_cb(uint32_t old_hw_mode_index,
|
||||
|
||||
/* update pm_conc_connection_list */
|
||||
policy_mgr_update_hw_mode_conn_info(context, num_vdev_mac_entries,
|
||||
vdev_mac_map,
|
||||
hw_mode);
|
||||
vdev_mac_map, hw_mode,
|
||||
num_mac_freq, mac_freq_range);
|
||||
|
||||
if (pm_ctx->mode_change_cb)
|
||||
pm_ctx->mode_change_cb();
|
||||
|
@@ -854,12 +854,136 @@ void policy_mgr_restore_deleted_conn_info(struct wlan_objmgr_psoc *psoc,
|
||||
info->vdev_id, conn_index);
|
||||
}
|
||||
|
||||
static bool
|
||||
policy_mgr_is_freq_range_5_6ghz(qdf_freq_t start_freq, qdf_freq_t end_freq)
|
||||
{
|
||||
if ((wlan_reg_is_5ghz_ch_freq(start_freq) ||
|
||||
wlan_reg_is_6ghz_chan_freq(start_freq)) &&
|
||||
(wlan_reg_is_5ghz_ch_freq(end_freq) ||
|
||||
wlan_reg_is_6ghz_chan_freq(end_freq)))
|
||||
return true;
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
static bool
|
||||
policy_mgr_is_freq_range_2ghz(qdf_freq_t start_freq, qdf_freq_t end_freq)
|
||||
{
|
||||
if (wlan_reg_is_24ghz_ch_freq(start_freq) &&
|
||||
wlan_reg_is_24ghz_ch_freq(end_freq))
|
||||
return true;
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_fill_curr_mac_2ghz_freq(uint32_t pdev_id,
|
||||
struct policy_mgr_pdev_mac_freq_map *freq,
|
||||
struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
{
|
||||
pm_ctx->hw_mode.cur_mac_freq_range[pdev_id].low_2ghz_freq =
|
||||
freq->start_freq;
|
||||
pm_ctx->hw_mode.cur_mac_freq_range[pdev_id].high_2ghz_freq =
|
||||
freq->end_freq;
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_fill_curr_mac_5ghz_freq(uint32_t pdev_id,
|
||||
struct policy_mgr_pdev_mac_freq_map *freq,
|
||||
struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
{
|
||||
pm_ctx->hw_mode.cur_mac_freq_range[pdev_id].low_5ghz_freq =
|
||||
freq->start_freq;
|
||||
pm_ctx->hw_mode.cur_mac_freq_range[pdev_id].high_5ghz_freq =
|
||||
freq->end_freq;
|
||||
}
|
||||
|
||||
void
|
||||
policy_mgr_fill_curr_mac_freq_by_hwmode(struct policy_mgr_psoc_priv_obj *pm_ctx,
|
||||
enum policy_mgr_mode mode_hw)
|
||||
{
|
||||
uint8_t i;
|
||||
struct policy_mgr_freq_range *cur_mac_freq, *hwmode_freq;
|
||||
|
||||
cur_mac_freq = pm_ctx->hw_mode.cur_mac_freq_range;
|
||||
hwmode_freq = pm_ctx->hw_mode.freq_range_caps[mode_hw];
|
||||
|
||||
for (i = 0; i < MAX_MAC; i++) {
|
||||
cur_mac_freq[i].low_2ghz_freq = hwmode_freq[i].low_2ghz_freq;
|
||||
cur_mac_freq[i].high_2ghz_freq = hwmode_freq[i].high_2ghz_freq;
|
||||
cur_mac_freq[i].low_5ghz_freq = hwmode_freq[i].low_5ghz_freq;
|
||||
cur_mac_freq[i].high_5ghz_freq = hwmode_freq[i].high_5ghz_freq;
|
||||
}
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_fill_legacy_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx,
|
||||
struct policy_mgr_hw_mode_params hw_mode)
|
||||
{
|
||||
enum policy_mgr_mode mode;
|
||||
|
||||
mode = hw_mode.dbs_cap ? MODE_DBS : MODE_SMM;
|
||||
policy_mgr_fill_curr_mac_freq_by_hwmode(pm_ctx, mode);
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_fill_curr_freq_by_pdev_freq(int32_t num_mac_freq,
|
||||
struct policy_mgr_pdev_mac_freq_map *freq,
|
||||
struct policy_mgr_psoc_priv_obj *pm_ctx,
|
||||
struct policy_mgr_hw_mode_params hw_mode)
|
||||
{
|
||||
uint32_t pdev_id, i;
|
||||
|
||||
for (i = 0; i < num_mac_freq; i++) {
|
||||
pdev_id = freq[i].pdev_id;
|
||||
|
||||
if (pdev_id >= MAX_MAC) {
|
||||
policy_mgr_debug("Invalid pdev id %d", pdev_id);
|
||||
return;
|
||||
}
|
||||
|
||||
policy_mgr_debug("pdev_id %d start freq %d end_freq %d",
|
||||
pdev_id, freq[i].start_freq,
|
||||
freq[i].end_freq);
|
||||
|
||||
if (policy_mgr_is_freq_range_2ghz(freq[i].start_freq,
|
||||
freq[i].end_freq))
|
||||
policy_mgr_fill_curr_mac_2ghz_freq(pdev_id,
|
||||
&freq[i],
|
||||
pm_ctx);
|
||||
else if (policy_mgr_is_freq_range_5_6ghz(freq[i].start_freq,
|
||||
freq[i].end_freq))
|
||||
policy_mgr_fill_curr_mac_5ghz_freq(pdev_id,
|
||||
&freq[i],
|
||||
pm_ctx);
|
||||
else
|
||||
policy_mgr_fill_legacy_freq_range(pm_ctx, hw_mode);
|
||||
}
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_update_curr_mac_freq(uint32_t num_mac_freq,
|
||||
struct policy_mgr_pdev_mac_freq_map *freq,
|
||||
struct policy_mgr_psoc_priv_obj *pm_ctx,
|
||||
struct policy_mgr_hw_mode_params hw_mode)
|
||||
{
|
||||
if (num_mac_freq && freq) {
|
||||
policy_mgr_fill_curr_freq_by_pdev_freq(num_mac_freq, freq,
|
||||
pm_ctx, hw_mode);
|
||||
return;
|
||||
}
|
||||
|
||||
policy_mgr_fill_legacy_freq_range(pm_ctx, hw_mode);
|
||||
}
|
||||
|
||||
/**
|
||||
* policy_mgr_update_hw_mode_conn_info() - Update connection
|
||||
* info based on HW mode
|
||||
* @num_vdev_mac_entries: Number of vdev-mac id entries that follow
|
||||
* @vdev_mac_map: Mapping of vdev-mac id
|
||||
* @hw_mode: HW mode
|
||||
* @num_mac_freq: number of Frequency Range
|
||||
* @freq_info: Pointer to Frequency Range
|
||||
*
|
||||
* Updates the connection info parameters based on the new HW mode
|
||||
*
|
||||
@@ -868,7 +992,9 @@ void policy_mgr_restore_deleted_conn_info(struct wlan_objmgr_psoc *psoc,
|
||||
void policy_mgr_update_hw_mode_conn_info(struct wlan_objmgr_psoc *psoc,
|
||||
uint32_t num_vdev_mac_entries,
|
||||
struct policy_mgr_vdev_mac_map *vdev_mac_map,
|
||||
struct policy_mgr_hw_mode_params hw_mode)
|
||||
struct policy_mgr_hw_mode_params hw_mode,
|
||||
uint32_t num_mac_freq,
|
||||
struct policy_mgr_pdev_mac_freq_map *freq_info)
|
||||
{
|
||||
uint32_t i, conn_index, found;
|
||||
struct policy_mgr_psoc_priv_obj *pm_ctx;
|
||||
@@ -905,6 +1031,9 @@ void policy_mgr_update_hw_mode_conn_info(struct wlan_objmgr_psoc *psoc,
|
||||
}
|
||||
}
|
||||
qdf_mutex_release(&pm_ctx->qdf_conc_list_lock);
|
||||
|
||||
policy_mgr_update_curr_mac_freq(num_mac_freq, freq_info, pm_ctx,
|
||||
hw_mode);
|
||||
policy_mgr_dump_connection_status_info(psoc);
|
||||
}
|
||||
|
||||
@@ -976,7 +1105,7 @@ void policy_mgr_pdev_set_hw_mode_cb(uint32_t status,
|
||||
policy_mgr_update_hw_mode_conn_info(context,
|
||||
num_vdev_mac_entries,
|
||||
vdev_mac_map,
|
||||
hw_mode);
|
||||
hw_mode, 0, NULL);
|
||||
if (pm_ctx->mode_change_cb)
|
||||
pm_ctx->mode_change_cb();
|
||||
|
||||
|
@@ -936,6 +936,22 @@ policy_mgr_update_mac_freq_info(struct wlan_objmgr_psoc *psoc,
|
||||
}
|
||||
}
|
||||
|
||||
void
|
||||
policy_mgr_dump_curr_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
{
|
||||
uint32_t i;
|
||||
struct policy_mgr_freq_range *freq_range;
|
||||
|
||||
freq_range = pm_ctx->hw_mode.cur_mac_freq_range;
|
||||
for (i = 0; i < MAX_MAC; i++)
|
||||
if (freq_range[i].low_2ghz_freq || freq_range[i].low_5ghz_freq)
|
||||
policymgr_nofl_info("PLCY_MGR_FREQ_RANGE_CUR: mac_id %d: 2Ghz: low %d high %d, 5Ghz: low %d high %d",
|
||||
i, freq_range[i].low_2ghz_freq,
|
||||
freq_range[i].high_2ghz_freq,
|
||||
freq_range[i].low_5ghz_freq,
|
||||
freq_range[i].high_5ghz_freq);
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_dump_freq_range_per_mac(struct policy_mgr_freq_range *freq_range,
|
||||
enum policy_mgr_mode hw_mode)
|
||||
@@ -953,21 +969,7 @@ policy_mgr_dump_freq_range_per_mac(struct policy_mgr_freq_range *freq_range,
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_dump_curr_freq_range(struct policy_mgr_freq_range *freq_range)
|
||||
{
|
||||
uint32_t i;
|
||||
|
||||
for (i = 0; i < MAX_MAC; i++)
|
||||
if (freq_range[i].low_2ghz_freq || freq_range[i].low_5ghz_freq)
|
||||
policymgr_nofl_info("PLCY_MGR_FREQ_RANGE_CUR: mac_id %d: 2Ghz: low %d high %d, 5Ghz: low %d high %d",
|
||||
i, freq_range[i].low_2ghz_freq,
|
||||
freq_range[i].high_2ghz_freq,
|
||||
freq_range[i].low_5ghz_freq,
|
||||
freq_range[i].high_5ghz_freq);
|
||||
}
|
||||
|
||||
static void
|
||||
policy_mgr_dump_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
policy_mgr_dump_hw_modes_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
{
|
||||
uint32_t i;
|
||||
struct policy_mgr_freq_range *freq_range;
|
||||
@@ -976,9 +978,13 @@ policy_mgr_dump_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
freq_range = pm_ctx->hw_mode.freq_range_caps[i];
|
||||
policy_mgr_dump_freq_range_per_mac(freq_range, i);
|
||||
}
|
||||
}
|
||||
|
||||
freq_range = pm_ctx->hw_mode.cur_mac_freq_range;
|
||||
policy_mgr_dump_curr_freq_range(freq_range);
|
||||
void
|
||||
policy_mgr_dump_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx)
|
||||
{
|
||||
policy_mgr_dump_hw_modes_freq_range(pm_ctx);
|
||||
policy_mgr_dump_curr_freq_range(pm_ctx);
|
||||
}
|
||||
|
||||
QDF_STATUS policy_mgr_update_hw_mode_list(struct wlan_objmgr_psoc *psoc,
|
||||
@@ -1068,6 +1074,11 @@ QDF_STATUS policy_mgr_update_hw_mode_list(struct wlan_objmgr_psoc *psoc,
|
||||
mac1_ss_bw_info, i, tmp->hw_mode_id, dbs_mode,
|
||||
sbs_mode);
|
||||
}
|
||||
|
||||
/*
|
||||
* Initializing Current frequency with SMM frequency.
|
||||
*/
|
||||
policy_mgr_fill_curr_mac_freq_by_hwmode(pm_ctx, MODE_SMM);
|
||||
policy_mgr_dump_freq_range(pm_ctx);
|
||||
|
||||
return QDF_STATUS_SUCCESS;
|
||||
@@ -3965,6 +3976,7 @@ void policy_mgr_dump_connection_status_info(struct wlan_objmgr_psoc *psoc)
|
||||
}
|
||||
qdf_mutex_release(&pm_ctx->qdf_conc_list_lock);
|
||||
|
||||
policy_mgr_dump_freq_range(pm_ctx);
|
||||
policy_mgr_validate_conn_info(psoc);
|
||||
}
|
||||
|
||||
@@ -5038,7 +5050,7 @@ QDF_STATUS policy_mgr_update_nan_vdev_mac_info(struct wlan_objmgr_psoc *psoc,
|
||||
|
||||
if (QDF_IS_STATUS_SUCCESS(status))
|
||||
policy_mgr_update_hw_mode_conn_info(psoc, 1, &vdev_mac_map,
|
||||
hw_mode);
|
||||
hw_mode, 0, NULL);
|
||||
|
||||
return status;
|
||||
}
|
||||
|
@@ -538,7 +538,9 @@ void policy_mgr_restore_deleted_conn_info(struct wlan_objmgr_psoc *psoc,
|
||||
void policy_mgr_update_hw_mode_conn_info(struct wlan_objmgr_psoc *psoc,
|
||||
uint32_t num_vdev_mac_entries,
|
||||
struct policy_mgr_vdev_mac_map *vdev_mac_map,
|
||||
struct policy_mgr_hw_mode_params hw_mode);
|
||||
struct policy_mgr_hw_mode_params hw_mode,
|
||||
uint32_t num_mac_freq,
|
||||
struct policy_mgr_pdev_mac_freq_map *freq_info);
|
||||
void policy_mgr_pdev_set_hw_mode_cb(uint32_t status,
|
||||
uint32_t cfgd_hw_mode_index,
|
||||
uint32_t num_vdev_mac_entries,
|
||||
@@ -679,6 +681,51 @@ enum policy_mgr_conc_next_action
|
||||
QDF_STATUS policy_mgr_reset_sap_mandatory_channels(
|
||||
struct policy_mgr_psoc_priv_obj *pm_ctx);
|
||||
|
||||
/**
|
||||
* policy_mgr_fill_curr_mac_freq_by_hwmode() - Fill Current Mac frequency with
|
||||
* the frequency range of the given Hw Mode
|
||||
*
|
||||
* @pm_ctx: Policy Mgr context
|
||||
* @mode_hw: Policy Mgr Hw mode
|
||||
*
|
||||
* Fill Current Mac frequency with the frequency range of the given Hw Mode
|
||||
*
|
||||
* Return: None
|
||||
*/
|
||||
void
|
||||
policy_mgr_fill_curr_mac_freq_by_hwmode(struct policy_mgr_psoc_priv_obj *pm_ctx,
|
||||
enum policy_mgr_mode mode_hw);
|
||||
|
||||
/**
|
||||
* policy_mgr_update_hw_mode_list() - Function to print every frequency range
|
||||
* for both MAC 0 and MAC1 for every Hw mode
|
||||
*
|
||||
* @pm_ctx: Policy Mgr context
|
||||
*
|
||||
* This function to Function to print every frequency range
|
||||
* for both MAC 0 and MAC1 for every Hw mode
|
||||
*
|
||||
* Return: void
|
||||
*
|
||||
*/
|
||||
void
|
||||
policy_mgr_dump_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx);
|
||||
|
||||
/**
|
||||
* policy_mgr_dump_curr_freq_range() - Function to print current frequency range
|
||||
* for both MAC 0 and MAC1
|
||||
*
|
||||
* @pm_ctx: Policy Mgr context
|
||||
*
|
||||
* This function to Function to print current frequency range
|
||||
* for both MAC 0 and MAC1 for every Hw mode
|
||||
*
|
||||
* Return: void
|
||||
*
|
||||
*/
|
||||
void
|
||||
policy_mgr_dump_curr_freq_range(struct policy_mgr_psoc_priv_obj *pm_ctx);
|
||||
|
||||
/**
|
||||
* policy_mgr_reg_chan_change_callback() - Callback to be
|
||||
* invoked by regulatory module when valid channel list changes
|
||||
|
@@ -896,7 +896,7 @@ QDF_STATUS cm_fw_roam_complete(struct cnx_mgr *cm_ctx, void *data)
|
||||
roam_synch_data->hw_mode_trans_ind.new_hw_mode_index,
|
||||
roam_synch_data->hw_mode_trans_ind.num_vdev_mac_entries,
|
||||
roam_synch_data->hw_mode_trans_ind.vdev_mac_map,
|
||||
psoc);
|
||||
0, NULL, psoc);
|
||||
|
||||
cm_check_and_set_sae_single_pmk_cap(psoc, vdev_id);
|
||||
|
||||
|
@@ -2147,6 +2147,11 @@ struct cm_roam_values_copy {
|
||||
#define MAX_PN_LEN 8
|
||||
#define MAX_KEY_LEN 32
|
||||
|
||||
/* MAX_FREQ_RANGE_NUM shouldn't exceed as only in case od SBS there will be 3
|
||||
* frequency ranges, For DBS, it will be 2. For SMM, it will be 1
|
||||
*/
|
||||
#define MAX_FREQ_RANGE_NUM 3
|
||||
|
||||
/**
|
||||
* struct cm_ho_fail_ind - ho fail indication to CM
|
||||
* @vdev_id: vdev id
|
||||
@@ -2169,6 +2174,18 @@ struct policy_mgr_vdev_mac_map {
|
||||
uint32_t mac_id;
|
||||
};
|
||||
|
||||
/**
|
||||
* struct policy_mgr_pdev_mac_freq_map - vdev id-mac id map
|
||||
* @pdev_id: Pdev id, macros starting with WMI_PDEV_ID_
|
||||
* @start_freq: Start Frequency in Mhz
|
||||
* @end_freq: End Frequency in Mhz
|
||||
*/
|
||||
struct policy_mgr_pdev_mac_freq_map {
|
||||
uint32_t pdev_id;
|
||||
qdf_freq_t start_freq;
|
||||
qdf_freq_t end_freq;
|
||||
};
|
||||
|
||||
/**
|
||||
* struct cm_hw_mode_trans_ind - HW mode transition indication
|
||||
* @old_hw_mode_index: Index of old HW mode
|
||||
@@ -2181,6 +2198,8 @@ struct cm_hw_mode_trans_ind {
|
||||
uint32_t new_hw_mode_index;
|
||||
uint32_t num_vdev_mac_entries;
|
||||
struct policy_mgr_vdev_mac_map vdev_mac_map[MAX_VDEV_SUPPORTED];
|
||||
uint32_t num_freq_map;
|
||||
struct policy_mgr_pdev_mac_freq_map mac_freq_map[MAX_FREQ_RANGE_NUM];
|
||||
};
|
||||
|
||||
/*
|
||||
|
@@ -495,6 +495,15 @@ static void lim_process_hw_mode_trans_ind(struct mac_context *mac, void *body)
|
||||
ind->vdev_mac_map[i].mac_id;
|
||||
}
|
||||
|
||||
param->num_freq_map = ind->num_freq_map;
|
||||
for (i = 0; i < param->num_freq_map; i++) {
|
||||
param->mac_freq_map[i].pdev_id =
|
||||
ind->mac_freq_map[i].pdev_id;
|
||||
param->mac_freq_map[i].start_freq =
|
||||
ind->mac_freq_map[i].start_freq;
|
||||
param->mac_freq_map[i].end_freq =
|
||||
ind->mac_freq_map[i].end_freq;
|
||||
}
|
||||
/* TODO: Update this HW mode info in any UMAC params, if needed */
|
||||
|
||||
msg.type = eWNI_SME_HW_MODE_TRANS_IND;
|
||||
|
@@ -268,7 +268,8 @@ static QDF_STATUS sme_process_hw_mode_trans_ind(struct mac_context *mac,
|
||||
policy_mgr_hw_mode_transition_cb(param->old_hw_mode_index,
|
||||
param->new_hw_mode_index,
|
||||
param->num_vdev_mac_entries,
|
||||
param->vdev_mac_map, mac->psoc);
|
||||
param->vdev_mac_map, param->num_freq_map, param->mac_freq_map,
|
||||
mac->psoc);
|
||||
|
||||
return QDF_STATUS_SUCCESS;
|
||||
}
|
||||
|
@@ -3897,6 +3897,37 @@ void wma_process_pdev_hw_mode_trans_ind(void *handle,
|
||||
wma->old_hw_mode_index, wma->new_hw_mode_index);
|
||||
}
|
||||
|
||||
static void
|
||||
wma_process_mac_freq_mapping(struct cm_hw_mode_trans_ind *hw_mode_trans_ind,
|
||||
WMI_PDEV_HW_MODE_TRANSITION_EVENTID_param_tlvs *param_buf)
|
||||
{
|
||||
uint32_t i, num_mac_freq;
|
||||
wmi_pdev_band_to_mac *mac_freq;
|
||||
|
||||
mac_freq = param_buf->mac_freq_mapping;
|
||||
num_mac_freq = param_buf->num_mac_freq_mapping;
|
||||
|
||||
if (!mac_freq) {
|
||||
wma_debug("mac_freq Null");
|
||||
return;
|
||||
}
|
||||
|
||||
if (!num_mac_freq || num_mac_freq > MAX_FREQ_RANGE_NUM) {
|
||||
wma_debug("num mac freq invalid %d", num_mac_freq);
|
||||
return;
|
||||
}
|
||||
|
||||
hw_mode_trans_ind->num_freq_map = num_mac_freq;
|
||||
for (i = 0; i < num_mac_freq; i++) {
|
||||
hw_mode_trans_ind->mac_freq_map[i].pdev_id =
|
||||
mac_freq[i].pdev_id;
|
||||
hw_mode_trans_ind->mac_freq_map[i].start_freq =
|
||||
mac_freq[i].start_freq;
|
||||
hw_mode_trans_ind->mac_freq_map[i].end_freq =
|
||||
mac_freq[i].end_freq;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* wma_pdev_hw_mode_transition_evt_handler() - HW mode transition evt handler
|
||||
* @handle: WMI handle
|
||||
@@ -3953,8 +3984,10 @@ static int wma_pdev_hw_mode_transition_evt_handler(void *handle,
|
||||
qdf_mem_free(hw_mode_trans_ind);
|
||||
return -EINVAL;
|
||||
}
|
||||
|
||||
wma_process_pdev_hw_mode_trans_ind(wma, wmi_event, vdev_mac_entry,
|
||||
hw_mode_trans_ind);
|
||||
wma_process_mac_freq_mapping(hw_mode_trans_ind, param_buf);
|
||||
/* Pass the message to PE */
|
||||
wma_send_msg(wma, SIR_HAL_PDEV_HW_MODE_TRANS_IND,
|
||||
(void *) hw_mode_trans_ind, 0);
|
||||
|
In neuem Issue referenzieren
Einen Benutzer sperren